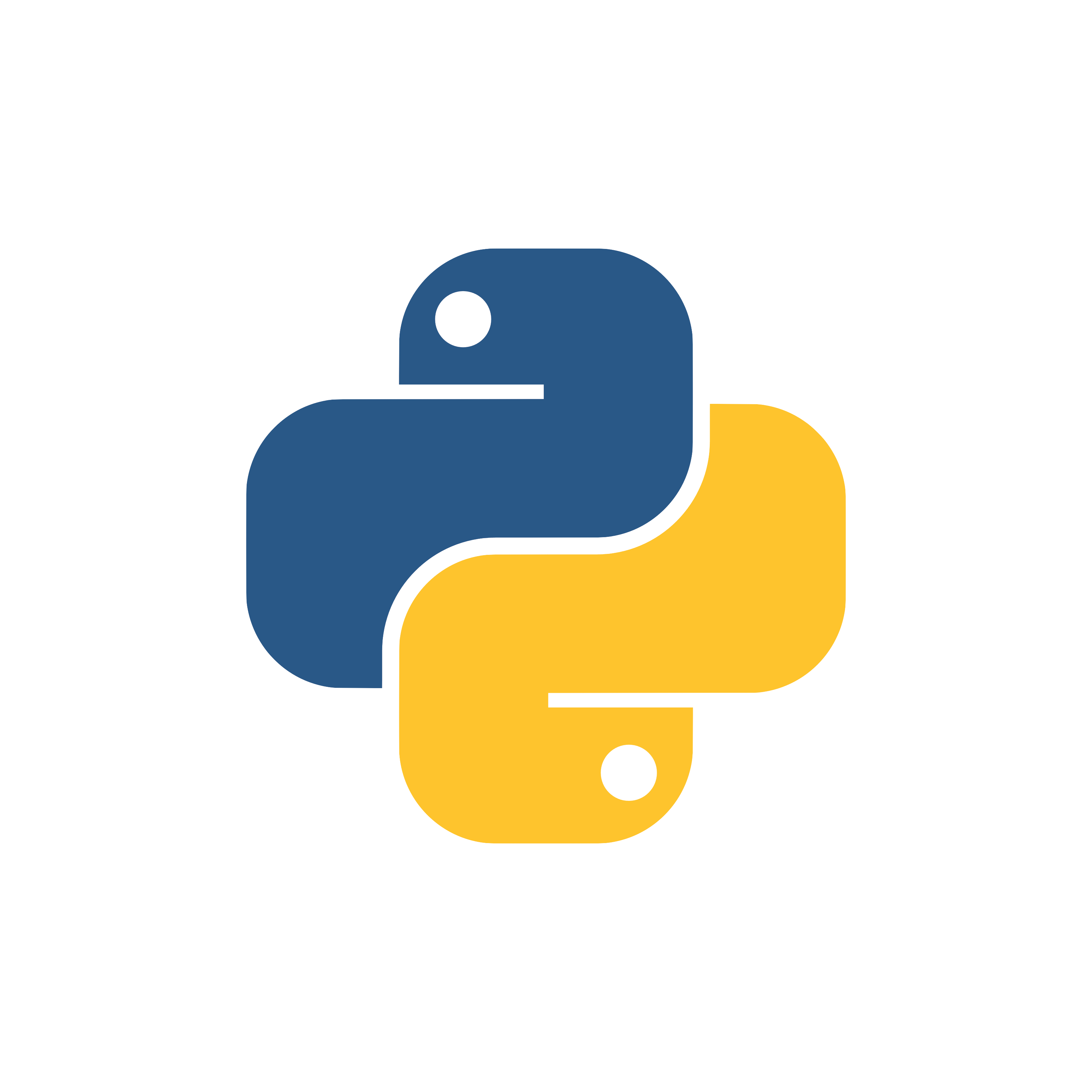
Python
- Python is an interpreted, high-level, general-purpose programming language.
- Created by Guido van Rossum and first released in 1991, Python has a design philosophy that emphasizes code readability, notably using significant whitespace.
- It provides constructs that enable clear programming on both small and large scales.
- Python is developed under an OSI-approved open source license, making it freely usable and distributable, even for commercial use.
- Python's license is administered by the Python Software Foundation.
- The Python interpreter and the extensive standard library are freely available in source or binary form for all major platforms from the Python web site, https://www.python.org/, and may be freely distributed.
Differences Between an Interpreter and a Compiler
We generally write a computer program using a high-level language. A high-level language is one that is understandable by us, humans. This is called source code.
However, a computer does not understand high-level language. It only understands the program written in 0's and 1's in binary, called the machine code.
To convert source code into machine code, we use either a compiler or an interpreter.
Both compilers and interpreters are used to convert a program written in a high-level language into machine code understood by computers. However, there are differences between how an interpreter and a compiler works.
Interpreter | Compiler |
---|---|
Translates program one statement at a time. | Scans the entire program and translates it as a whole into machine code. |
Interpreters usually take less amount of time to analyze the source code. However, the overall execution time is comparatively slower than compilers. | Compilers usually take a large amount of time to analyze the source code. However, the overall execution time is comparatively faster than interpreters. |
No Object Code is generated, hence are memory efficient. | Generates Object Code which further requires linking, hence requires more memory. |
Programming languages like JavaScript, Python, Ruby use interpreters. | Programming languages like C, C++, Java use compilers. |
Working of Compiler and Interpreter
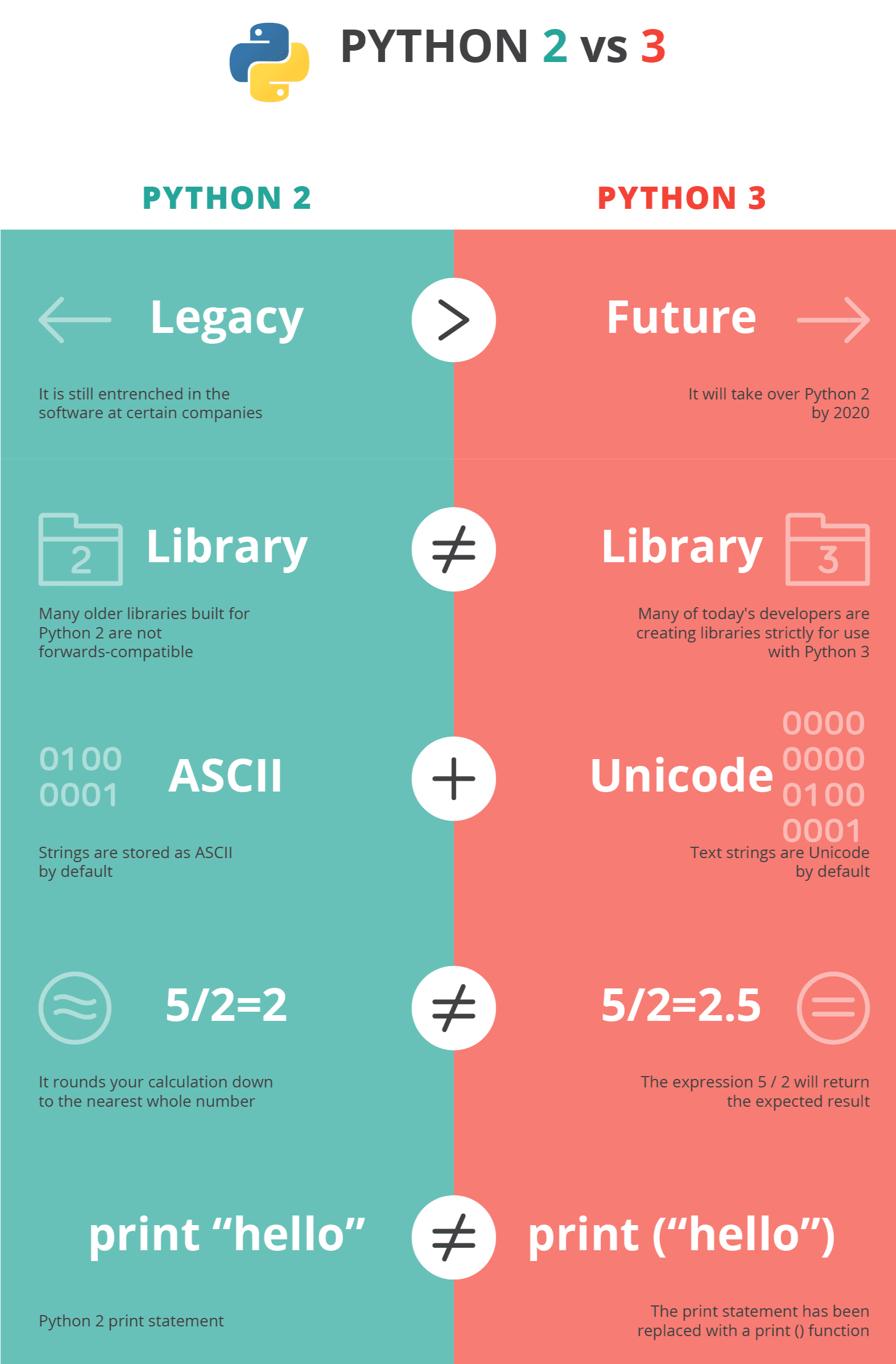
Some advantages of Python
Python is simple to use, but it is a real programming language, offering much more structure and support for large programs than shell scripts or batch files can offer. On the other hand, Python also offers much more error checking than C, and, being a very-high-level language, it has high-level data types built in, such as flexible arrays and dictionaries. Because of its more general data types Python is applicable to a much larger problem domain than Awk or even Perl, yet many things are at least as easy in Python as in those languages.
Python allows you to split your program into modules that can be reused in other Python programs. It comes with a large collection of standard modules that you can use as the basis of your programs — or as examples to start learning to program in Python. Some of these modules provide things like file I/O, system calls, sockets, and even interfaces to graphical user interface toolkits like Tk.
Python is an interpreted language, which can save you considerable time during program development because no compilation and linking is necessary. The interpreter can be used interactively, which makes it easy to experiment with features of the language, to write throw-away programs, or to test functions during bottom-up program development. It is also a handy desk calculator.
Python enables programs to be written compactly and readably. Programs written in Python are typically much shorter than equivalent C, C++, or Java programs, for several reasons:
the high-level data types allow you to express complex operations in a single statement;
statement grouping is done by indentation instead of beginning and ending brackets;
no variable or argument declarations are necessary.
Python is extensible: if you know how to program in C it is easy to add a new built-in function or module to the interpreter, either to perform critical operations at maximum speed, or to link Python programs to libraries that may only be available in binary form (such as a vendor-specific graphics library). Once you are really hooked, you can link the Python interpreter into an application written in C and use it as an extension or command language for that application.
Programming-Language features of Python
- A variety of basic data types are available: numbers (floating point, complex, and unlimited-length long integers), strings (both ASCII and Unicode), lists, and dictionaries.
- Python supports object-oriented programming with classes and multiple inheritance.
- Code can be grouped into modules and packages.
- The language supports raising and catching exceptions, resulting in cleaner error handling.
- Data types are strongly and dynamically typed. Mixing incompatible types (e.g. attempting to add a string and a number) causes an exception to be raised, so errors are caught sooner.
- Python contains advanced programming features such as generators and list comprehensions.
- Python's automatic memory management frees you from having to manually allocate and free memory in your code.
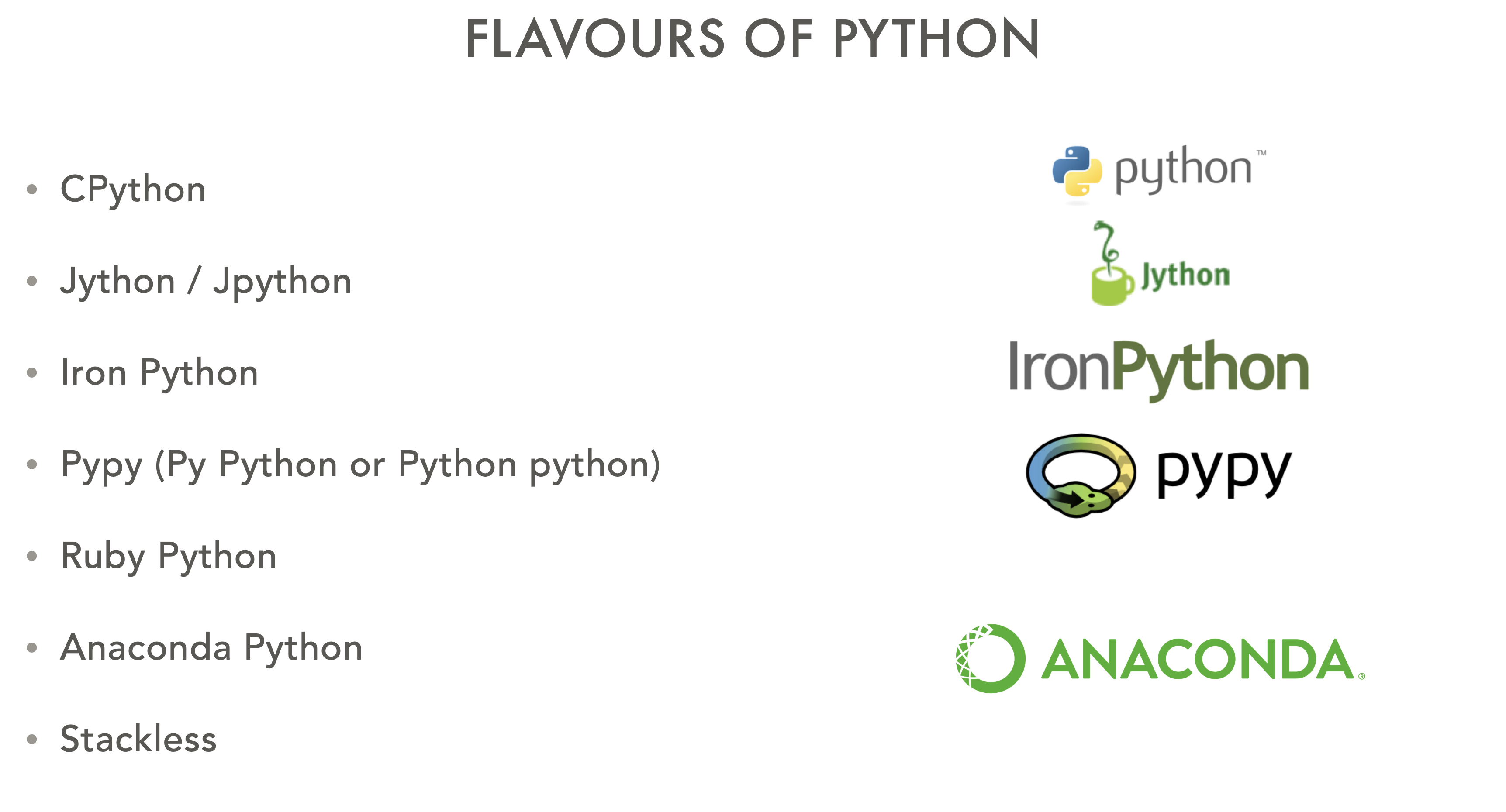
Installing Python 3
Python 3 come installed out of the box in Linux. Only we have to install a few more packages to make it more complete. We run the following command in Ubuntu to install the remaining packages.
sudo apt install python3-pip python3-venv python3-dev python-is-python3
Your first Python Program
Now that we have Python up and running, we can write our first Python program.
Let's create a very simple program called Hello World. A "Hello, World!" is a simple program that outputs Hello, World! on the screen. Since it's a very simple program, it's often used to introduce a new programming language to beginners.
Type the following code in any text editor or an IDE and save it as hello_world.py
print("Hello, world!")
Then, run the file by typing the following command:
(For Linux and Mac)
python3 hello_world.py
(For Windows)
python3 hello_world.py
This line instructs your Python interpreter to execute the command you just wrote. You should see the correct output
Using the Python Interpreter
The Python interpreter is usually installed as /usr/local/bin/python3.11 on those machines where it is available; putting /usr/local/bin in your Unix shell’s search path makes it possible to start it by typing the command: python3 to the shell.
On Windows machines where you have installed Python from the Microsoft Store, the python3.11
command will be available. If you have the py.exe launcher installed, you can use the py
command.
Typing an end-of-file character (Control-D on Unix, Control-Z on Windows) at the primary prompt causes the interpreter to exit with a zero exit status. If that doesn’t work, you can exit the interpreter by typing the following command: quit()
.
Interactive Mode
When commands are read from a tty, the interpreter is said to be in interactive mode. In this mode it prompts for the next command with the primary prompt, usually three greater-than signs (>>>
); for continuation lines it prompts with the secondary prompt, by default three dots (...
). The interpreter prints a welcome message stating its version number and a copyright notice before printing the first prompt:
python3.11 Python 3.11 (default, April 4 2021, 09:25:04) [GCC 10.2.0] on linux Type "help", "copyright", "credits" or "license" for more information. >>>
Declaration of Variables
Unlike other programming languages, Python has no command for declaring a variable.
A variable is created the moment you first assign a value to it.
A variable can have a short name (like x and y) or a more descriptive name (age, carname, total_volume).
Rules for Python variables:
- A variable name must start with a letter or the underscore character
- A variable name cannot start with a number
- A variable name can only contain alpha-numeric characters and underscores (A-z, 0-9, and _ )
- Variable names are case-sensitive (age, Age and AGE are three different variables)
Example
Try to guess the output
a = 'Hi There'
b = 2.22
c = 0xFF
print(a)
print(b)
print(c)
How do we find out the data type of each variable?
Hint:- We use a function for that.
a = 'Hi There'
b = 2.22
c = 0xFF
print(type(a))
print(type(b))
print(type(c))
Python Literals
Literals are representations of fixed values in a program. They can be numbers, characters, or strings, etc. For example, 'Hello, World!'
, 12
, 23.0
, 'C'
, etc.
Literals are often used to assign values to variables or constants. For example,
site_name = 'santechz.com'
In the above expression, site_name is a variable, and 'santechz.com'
is a literal.
Python Numeric Literals
Numeric Literals are immutable (unchangeable). Numeric literals can belong to 3 different numerical types: Integer
, Float
, and Complex
.
Type | Example | Remarks |
---|---|---|
Decimal | 5, 10, -68 | Regular numbers. |
Binary | 0b101, 0b11 | Start with 0b. |
Octal | 0o13 | Start with 0o. |
Hexadecimal | 0x13 | Start with 0x. |
Floating-point Literal | 10.5, 3.14 | Containing floating decimal points. |
Complex Literal | 6 + 9j | Numerals in the form a + bj , where a is real and b is imaginary part |
Python Boolean Literals
There are two boolean literals: True
and False
.
For example,
result1 = True
Here, True
is a boolean literal assigned to result1.
String and Character Literals in Python
Character literals are unicode characters enclosed in a quote. For example,
some_character = 'S'
Here, S
is a character literal assigned to some_character.
Similarly, String literals are sequences of Characters enclosed in quotation marks.
For example,
some_string = 'Python is fun'
Here, 'Python is fun' is a string literal assigned to some_string.
Special Literal in Python
Python contains one special literal None
. We use it to specify a null variable. For example,
value = None
print(value)
# Output: None
Here, we get None
as an output as the value variable has no value assigned to it.
Comments
Comments are lines that exist in computer programs that are ignored by compilers and interpreters. Including comments in programs makes code more readable for humans as it provides some information or explanation about what each part of a program is doing.
Depending on the purpose of your program, comments can serve as notes to yourself or reminders, or they can be written with the intention of other programmers being able to understand what your code is doing.
Comments in Python begin with a hash mark (#) and whitespace character and continue to the end of the line.
A Python multiline comment consists of a group of text enclosed in a delimiter (""") at each end. To avoid syntax errors, make sure to indent the first """ correctly. These are used when the comment content fits into multiple lines.
Example:
# Print Python to console
print("Python")
"""
This is a multiline comment
This is the next line.
"""
print("Language")
input()
Python provides numerous built-in functions that are readily available to us at the Python prompt.
In Python, we have the input() function to allow this.
The syntax for input() is :
input(<prompt>)
Example:
a=input('Enter a number')
print(a)
print()
We use the print() function to output data to the standard output device (screen).
The syntax of the print() function is
print(objects, sep=' ', end='\n', file=sys.stdout, flush=False)
object - value(s) to be printed
sep (optional) - allows us to separate multiple objects inside print().
end (optional) - allows us to add add specific values like new line "\n", tab "\t"
file (optional) - where the values are printed. It's default value is sys.stdout (screen)
flush (optional) - boolean specifying if the output is flushed or buffered. Default: False
Example:
print(1,2,3,4)
# Output: 1 2 3 4
print(1,2,3,4,sep='*')
# Output: 1*2*3*4
print(1,2,3,4,sep='#',end='&')
# Output: 1#2#3#4&
Formatting Strings
- Python uses C-style string formatting to create new, formatted strings.
- The "%" operator is used to format a set of variables enclosed in a "tuple" (a fixed size list), together with a format string, which contains normal text together with "argument specifiers", special symbols like "%s" and “%d"
- Sometimes we would like to format our output to make it look attractive. This can be done by using the format() method.
- The curly braces {} are used as placeholders for the format function.
Example:
name = input('Enter your name: ')
age = input('Enter your age: ')
age = int(age)
result1 = 'Name: %s Age: %d' % (name,age)
result2 = 'Your Name is {}. You are {} years old.'.format(name,age)
result3 = 'You are {1} years old and your name is {0}.'.format(name,age)
print(result1,result2,result3,sep='\n')
Now Guess the Output
Indenting Code
A block is a group of statements in a program or script. Usually it consists of at least one statement and of declarations for the block, depending on the programming or scripting language. A language which allows grouping with blocks, is called a block structured language.
Python programs get structured through indentation, i.e. code blocks are defined by their indentation.
This principle makes it easier to read and understand other people's Python code.
All statements with the same distance to the right belong to the same block of code, i.e. the statements within a block line up vertically. The block ends at a line less indented or the end of the file. If a block has to be more deeply nested, it is simply indented further to the right.
Python Keywords
We cannot use a keyword as a variable name, function name or any other identifier. They are used to define the syntax and structure of the Python language. In Python, keywords are case sensitive.
The following identifiers are used as reserved words, or keywords of the language, and cannot be used as ordinary identifiers. They must be spelled exactly as written here:
False await else import pass
None break except in raise
True class finally is return
and continue for lambda try
as def from nonlocal while
assert del global not with
async elif if or yield
Built-in Data Types
In programming, data type is an important concept.
Variables can store data of different types, and different types can do different things.
Python has the following data types built-in by default, in these categories:
Text Type: | str |
Numeric Types: | int , float , complex |
Sequence Types: | list , tuple , range |
Mapping Type: | dict |
Set Types: | set , frozenset |
Boolean Type: | bool |
Binary Types: | bytes , bytearray , memoryview |
None Type: | NoneType |
Escape Characters
To insert characters that are illegal in a string, use an escape character.
An escape character is a backslash \ followed by the character you want to insert.
An example of an illegal character is a double quote inside a string that is surrounded by double quotes:
Example:
You will get an error if you use double quotes inside a string that is surrounded by double quotes:
txt = "We are the so-called "Vikings" from the north."
To fix this problem, use the escape character \":
The escape character allows you to use double quotes when you normally would not be allowed:
txt = "We are the so-called \"Vikings\" from the north."
Other escape characters used in Python:
Code |
Result |
\' |
Single Quote |
\\ |
Backslash |
\n |
New Line |
\r |
Carriage Return |
\t |
Tab |
\b |
Backspace |
\f |
Form Feed |
\ooo |
Octal value |
\xhh |
Hex value |
Various Operators in Python
Arithmetic Operators
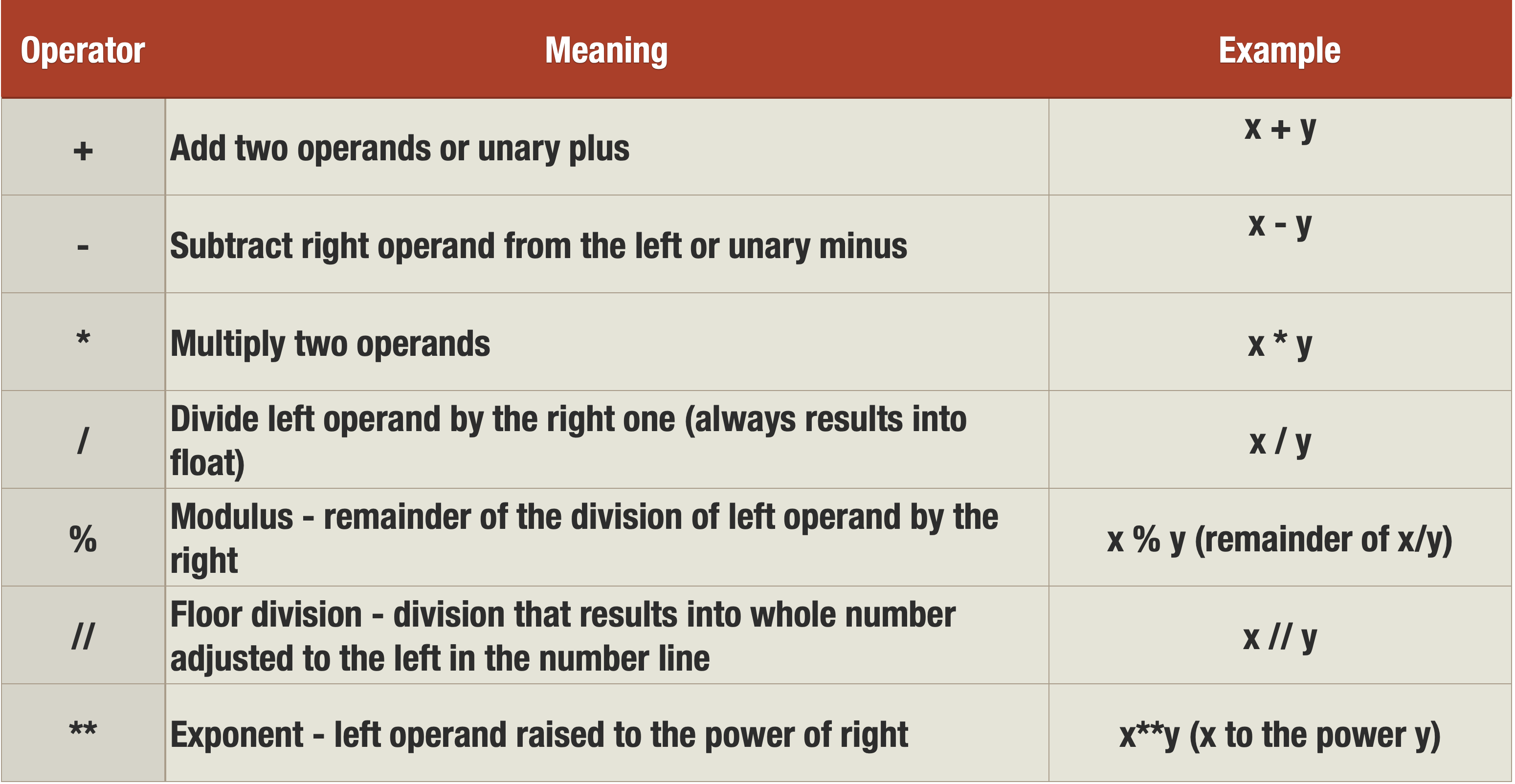
Comparison Operators
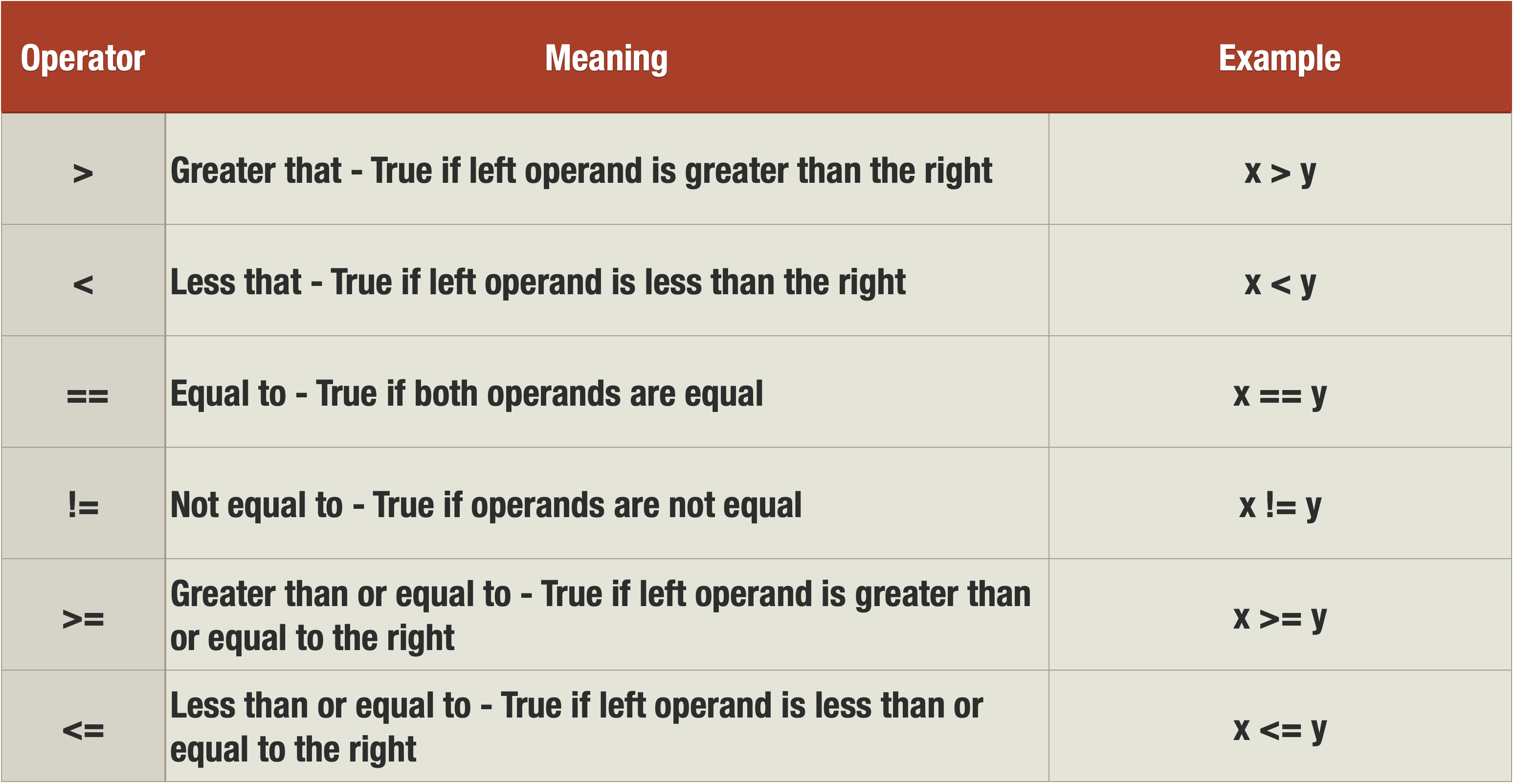
Bitwise Operators
In this example: - x=20 (0001 0100) and y=12 (0000 1100)
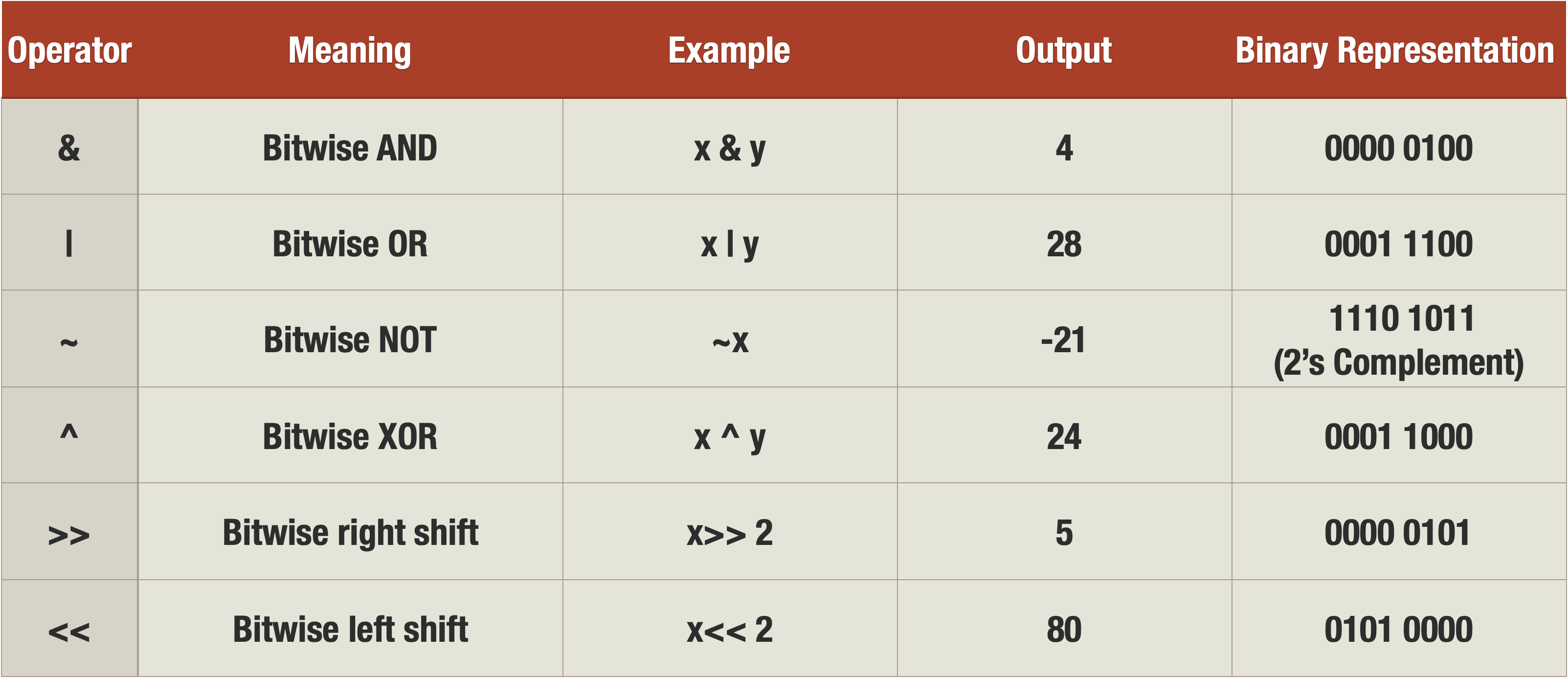
Assignment Operators in Python
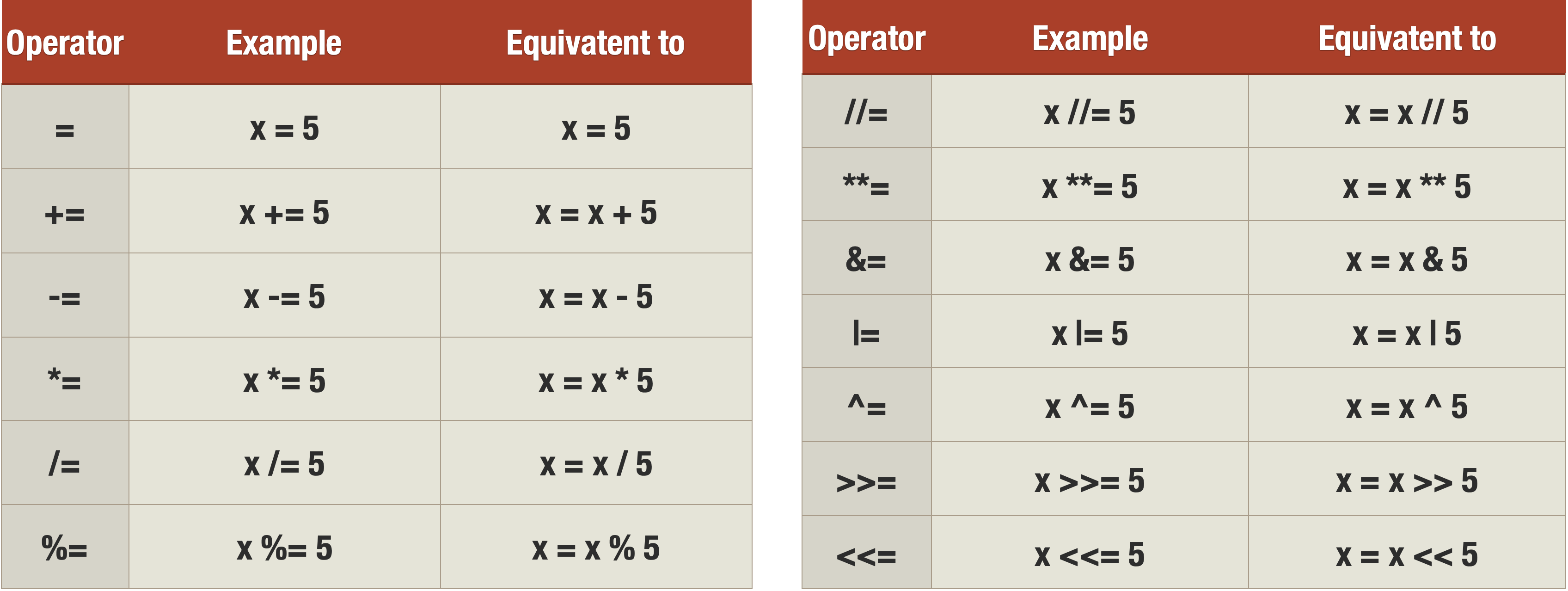
Logical Operators
Identity Operators
Python Type Conversion
In programming, type conversion is the process of converting data of one type to another. For example: converting int
data to str
.
There are two types of type conversion in Python.
- Implicit Conversion - automatic type conversion
- Explicit Conversion - manual type conversion
Python Implicit Type Conversion
In certain situations, Python automatically converts one data type to another. This is known as implicit type conversion.
Example 1: Converting integer to float
Let's see an example where Python promotes the conversion of the lower data type (integer) to the higher data type (float) to avoid data loss.
integer_number = 123
float_number = 1.23
new_number = integer_number + float_number
# display new value and resulting data type
print("Value:",new_number)
print("Data Type:",type(new_number))
Output:
Value: 124.23
Data Type: <class 'float'>
In the above example, we have created two variables: integer_number and float_numberof int
and float
type respectively.
Then we added these two variables and stored the result in new_number.
As we can see new_number has value 124.23 and is of the float
data type.
It is because Python always converts smaller data types to larger data types to avoid the loss of data.
Note:
- We get
TypeError
, if we try to addstr
andint
. For example,'12' + 23
. Python is not able to use Implicit Conversion in such conditions. - Python has a solution for these types of situations which is known as Explicit Conversion.
Explicit Type Conversion
In Explicit Type Conversion, users convert the data type of an object to required data type.
We use the built-in functions like int()
, float()
, str()
, etc to perform explicit type conversion.
This type of conversion is also called typecasting because the user casts (changes) the data type of the objects.
Example 2: Addition of string and integer Using Explicit Conversion
num_string = '12'
num_integer = 23
print("Data type of num_string before Type Casting:",type(num_string))
# explicit type conversion
num_string = int(num_string)
print("Data type of num_string after Type Casting:",type(num_string))
num_sum = num_integer + num_string
print("Sum:",num_sum)
print("Data type of num_sum:",type(num_sum))
Output:
Data type of num_string before Type Casting: <class 'str'>
Data type of num_string after Type Casting: <class 'int'>
Sum: 35
Data type of num_sum: <class 'int'>
In the above example, we have created two variables: num_string and num_integer with str
and int
type values respectively. Notice the code,
num_string = int(num_string)
Here, we have used int()
to perform explicit type conversion of num_string to integer type.
After converting num_string to an integer value, Python is able to add these two variables.
Finally, we got the num_sum value i.e 35 and data type to be int
.
Key Points to Remember
- Type Conversion is the conversion of an object from one data type to another data type.
- Implicit Type Conversion is automatically performed by the Python interpreter.
- Python avoids the loss of data in Implicit Type Conversion.
- Explicit Type Conversion is also called Type Casting, the data types of objects are converted using predefined functions by the user.
- In Type Casting, loss of data may occur as we enforce the object to a specific data type.